evren pehlivan
30 Temmuz 2018 Pazartesi
evren pehlivan ASP.NET Web Forms ile Web API kullanma
ASP.NET Web Forms ile Web API kullanma
tarafından Mike Wasson
ASP.NET Web API, ASP.NET MVC ile paketlenmiştir olsa da, Web API'si için geleneksel bir ASP.NET Web Forms uygulaması eklemek kolay bir işlemdir. Bu öğreticide, adım adım açıklanmaktadır.
Genel Bakış
Bir Web Forms uygulaması'nda Web API'sini kullanmak için iki ana adım vardır:
- Türetilen bir Web API denetleyicisi ekleme ApiController sınıfı.
- Bir rota tablosuna ekleme uygulama_Başlat yöntemi.
Web Forms projesi oluşturun
Visual Studio'yu başlatın ve seçin yeni proje gelen Başlat sayfası. Veya dosya menüsünde yeni ardından proje.
İçinde şablonları bölmesinde yüklü şablonlar genişletin Visual C# düğümü. Altında Visual C# seçin Web. Proje şablonları listesinde seçin ASP.NET Web Forms uygulaması. Proje için bir ad girin ve tıklayın Tamam.
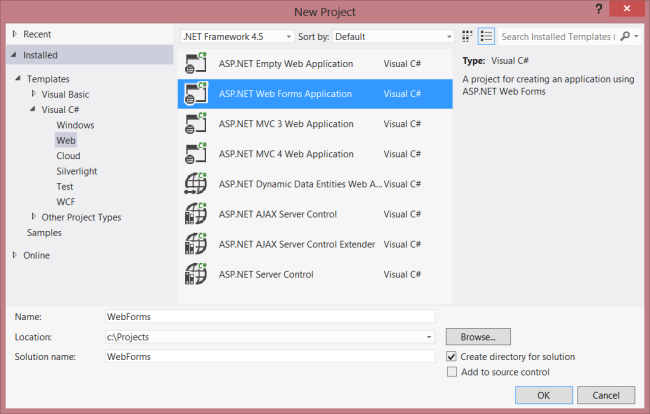
Model ve denetleyici oluşturma
Bu öğreticide aynı model ve denetleyici sınıflar olarak Başlarken öğretici.
İlk olarak, bir model sınıfı ekleyin. İçinde Çözüm Gezgini, projeye sağ tıklayıp seçin sınıfı Ekle. ' % S'sınıfı ürün adı ve aşağıdaki uygulama ekleyin:
C#
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
public string Category { get; set; }
}
Ardından, bir Web API denetleyicisi proje, A ekleyin. denetleyicisi Web API'si için HTTP isteklerini işleyen nesne.
İçinde Çözüm Gezgini, projeye sağ tıklayın. Seçin Add New Item.
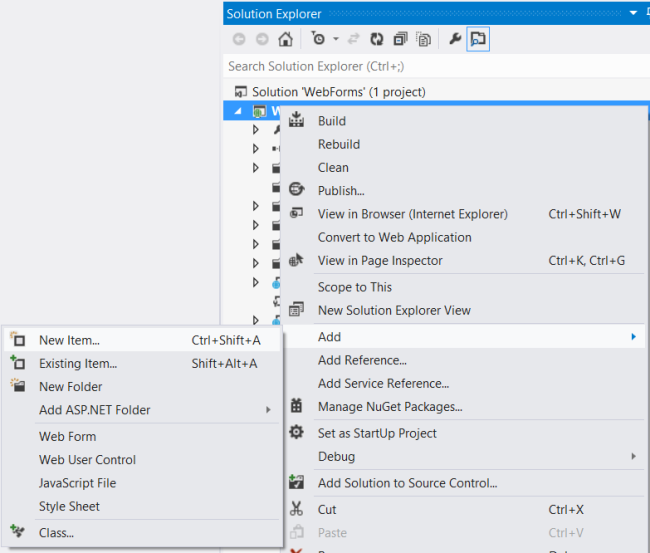
Altında yüklü şablonlar, genişletme Visual C# seçip Web. Şablonlar listesinden seçip Web API denetleyici sınıfı.Denetleyici "ProductsController" adını verin ve tıklayın Ekle.

Yeni Öğe Ekle Sihirbazı ProductsController.cs adlı bir dosya oluşturur. Sihirbaz dahil yöntemleri silin ve aşağıdaki yöntemleri ekleyin:
C#
namespace WebForms
{
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
public class ProductsController : ApiController
{
Product[] products = new Product[]
{
new Product { Id = 1, Name = "Tomato Soup", Category = "Groceries", Price = 1 },
new Product { Id = 2, Name = "Yo-yo", Category = "Toys", Price = 3.75M },
new Product { Id = 3, Name = "Hammer", Category = "Hardware", Price = 16.99M }
};
public IEnumerable<Product> GetAllProducts()
{
return products;
}
public Product GetProductById(int id)
{
var product = products.FirstOrDefault((p) => p.Id == id);
if (product == null)
{
throw new HttpResponseException(HttpStatusCode.NotFound);
}
return product;
}
public IEnumerable<Product> GetProductsByCategory(string category)
{
return products.Where(
(p) => string.Equals(p.Category, category,
StringComparison.OrdinalIgnoreCase));
}
}
}
Bu denetleyicideki kod hakkında daha fazla bilgi için bkz. Başlarken öğretici.
Yönlendirme bilgileri
Ardından, URI yolu şekilde ekleyeceğiz, bir URI'leri formun "/API'si/ürünler/" denetleyiciye yönlendirilir.
İçinde Çözüm Gezgini, Global.asax Global.asax.cs arka plan kod dosyasını açmak için çift tıklayın. Aşağıdaki kullanarak deyimi.
C#
using System.Web.Http;
Ardından aşağıdaki kodu ekleyin uygulama_Başlat yöntemi:
C#
RouteTable.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = System.Web.Http.RouteParameter.Optional }
);
Yönlendirme tabloları hakkında daha fazla bilgi için bkz. ASP.NET Web API'de yönlendirme.
İstemci tarafı AJAX ekleme
Tüm web istemcilerin erişebileceği bir API oluşturmak için gereken budur. Şimdi, API'yi çağırmak için jQuery kullanan bir HTML sayfası ekleyelim.
Ana sayfanıza emin olun (örneğin, Site.Master) içeren bir
ContentPlaceHolder
ile ID="HeadContent"
:
HTML
<asp:ContentPlaceHolder runat="server" ID="HeadContent"></asp:ContentPlaceHolder>
Default.aspx dosyasını açın. Ana içerik bölümü Demirbaş metni gösterildiği gibi değiştirin:
aspx
<%@ Page Title="Home Page" Language="C#" MasterPageFile="~/Site.Master"
AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="WebForms._Default" %>
<asp:Content ID="HeaderContent" runat="server" ContentPlaceHolderID="HeadContent">
</asp:Content>
<asp:Content ID="BodyContent" runat="server" ContentPlaceHolderID="MainContent">
<h2>Products</h2>
<table>
<thead>
<tr><th>Name</th><th>Price</th></tr>
</thead>
<tbody id="products">
</tbody>
</table>
</asp:Content>
Ardından, jQuery kaynak dosyasına bir başvuru ekleyin
HeaderContent
bölümü:
aspx
<asp:Content ID="HeaderContent" runat="server" ContentPlaceHolderID="HeadContent">
<script src="Scripts/jquery-1.10.2.min.js" type="text/javascript"></script>
</asp:Content>
Not: Kolayca betik başvurusu dosyasından sürükleyip bırakarak ekleyebilirsiniz Çözüm Gezgini içine kod düzenleyicisi penceresi.
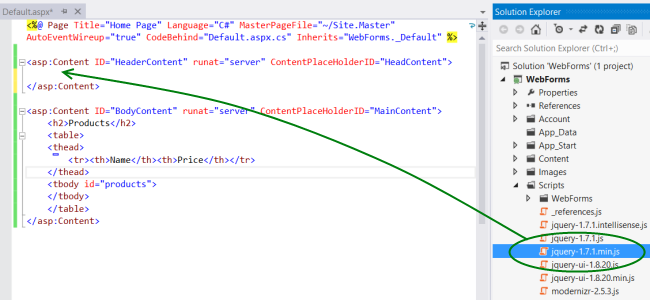
Aşağıdaki komut dosyası bloğu jQuery komut dosyası etiketi ekleyin:
HTML
<script type="text/javascript">
function getProducts() {
$.getJSON("api/products",
function (data) {
$('#products').empty(); // Clear the table body.
// Loop through the list of products.
$.each(data, function (key, val) {
// Add a table row for the product.
var row = '<td>' + val.Name + '</td><td>' + val.Price + '</td>';
$('<tr/>', { html: row }) // Append the name.
.appendTo($('#products'));
});
});
}
$(document).ready(getProducts);
</script>
Belge yüklendiğinde bu betik bir AJAX isteğinde bulunur. "API/ürünleri". İstek, JSON biçiminde ürünlerin listesini döndürür. Betik, ürün bilgisi HTML tablosuna ekler.
Uygulamayı çalıştırdığınızda, şu şekilde görünmelidir:
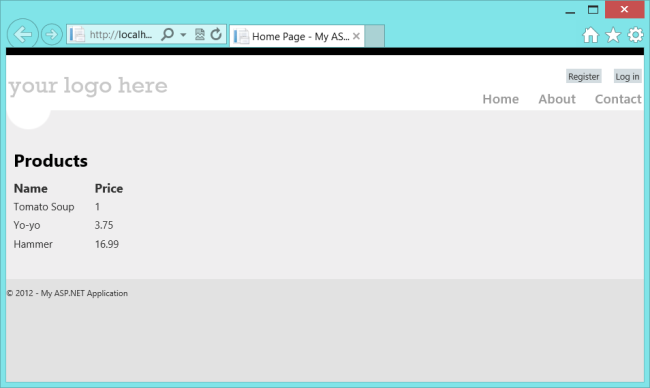
evren pehlivan
ekrem pehlivan
evren pehlivan
Use ASP.NET Core 2 to create durable and cross-platform web APIs through a series of applied, practical scenarios. Examples in this book help you build APIs that are fast and scalable. You'll progress from the basics of the framework through to solving the complex problems encountered in implementing secure RESTful services. The book is packed full of examples showing how Microsoft's ground-up rewrite of ASP.NET Core 2 enables native cross-platform applications that are fast and modular, allowing your cloud-ready server applications to scale as your business grows.
Major topics covered in the book include the fundamentals and core concepts of ASP.NET Core 2. You'll learn about building RESTful APIs with the MVC pattern using proven best practices and following the six principles of REST. Examples in the book help in learning to develop world-class web APIs and applications that can run on any platform, including Windows, Linux, and MacOS. You can even deploy to Microsoft Azure and automate your delivery by implementing Continuous Integration and Continuous Deployment pipelines.
Incorporate automated API tooling such as Swagger from the OpenAPI specification; Standardize query and response formats using Facebook's GraphQL query language; Implement security by applying authentication and authorization using ASP.NET Identity; Ensure the safe storage of sensitive data using the data protection stack; Create unit and integration tests to guarantee code quality.
Use ASP.NET Core 2 to create durable and cross-platform web APIs through a series of applied, practical scenarios. Examples in this book help you build APIs that are fast and scalable. You'll progress from the basics of the framework through to solving the complex problems encountered in implementing secure RESTful services. The book is packed full of examples showing how Microsoft's ground-up rewrite of ASP.NET Core 2 enables native cross-platform applications that are fast and modular, allowing your cloud-ready server applications to scale as your business grows.
Major topics covered in the book include the fundamentals and core concepts of ASP.NET Core 2. You'll learn about building RESTful APIs with the MVC pattern using proven best practices and following the six principles of REST. Examples in the book help in learning to develop world-class web APIs and applications that can run on any platform, including Windows, Linux, and MacOS. You can even deploy to Microsoft Azure and automate your delivery by implementing Continuous Integration and Continuous Deployment pipelines.
Incorporate automated API tooling such as Swagger from the OpenAPI specification; Standardize query and response formats using Facebook's GraphQL query language; Implement security by applying authentication and authorization using ASP.NET Identity; Ensure the safe storage of sensitive data using the data protection stack; Create unit and integration tests to guarantee code quality.
Use ASP.NET Core 2 to create durable and cross-platform web APIs through a series of applied, practical scenarios. Examples in this book help you build APIs that are fast and scalable. You'll progress from the basics of the framework through to solving the complex problems encountered in implementing secure RESTful services. The book is packed full of examples showing how Microsoft's ground-up rewrite of ASP.NET Core 2 enables native cross-platform applications that are fast and modular, allowing your cloud-ready server applications to scale as your business grows.
Major topics covered in the book include the fundamentals and core concepts of ASP.NET Core 2. You'll learn about building RESTful APIs with the MVC pattern using proven best practices and following the six principles of REST. Examples in the book help in learning to develop world-class web APIs and applications that can run on any platform, including Windows, Linux, and MacOS. You can even deploy to Microsoft Azure and automate your delivery by implementing Continuous Integration and Continuous Deployment pipelines.
Incorporate automated API tooling such as Swagger from the OpenAPI specification; Standardize query and response formats using Facebook's GraphQL query language; Implement security by applying authentication and authorization using ASP.NET Identity; Ensure the safe storage of sensitive data using the data protection stack; Create unit and integration tests to guarantee code quality.
evren pehlivan
Use ASP.NET Core 2 to create durable and cross-platform web APIs through a series of applied, practical scenarios. Examples in this book help you build APIs that are fast and scalable. You'll progress from the basics of the framework through to solving the complex problems encountered in implementing secure RESTful services. The book is packed full of examples showing how Microsoft's ground-up rewrite of ASP.NET Core 2 enables native cross-platform applications that are fast and modular, allowing your cloud-ready server applications to scale as your business grows.
Major topics covered in the book include the fundamentals and core concepts of ASP.NET Core 2. You'll learn about building RESTful APIs with the MVC pattern using proven best practices and following the six principles of REST. Examples in the book help in learning to develop world-class web APIs and applications that can run on any platform, including Windows, Linux, and MacOS. You can even deploy to Microsoft Azure and automate your delivery by implementing Continuous Integration and Continuous Deployment pipelines.
Incorporate automated API tooling such as Swagger from the OpenAPI specification; Standardize query and response formats using Facebook's GraphQL query language; Implement security by applying authentication and authorization using ASP.NET Identity; Ensure the safe storage of sensitive data using the data protection stack; Create unit and integration tests to guarantee code quality.
Use ASP.NET Core 2 to create durable and cross-platform web APIs through a series of applied, practical scenarios. Examples in this book help you build APIs that are fast and scalable. You'll progress from the basics of the framework through to solving the complex problems encountered in implementing secure RESTful services. The book is packed full of examples showing how Microsoft's ground-up rewrite of ASP.NET Core 2 enables native cross-platform applications that are fast and modular, allowing your cloud-ready server applications to scale as your business grows.
Major topics covered in the book include the fundamentals and core concepts of ASP.NET Core 2. You'll learn about building RESTful APIs with the MVC pattern using proven best practices and following the six principles of REST. Examples in the book help in learning to develop world-class web APIs and applications that can run on any platform, including Windows, Linux, and MacOS. You can even deploy to Microsoft Azure and automate your delivery by implementing Continuous Integration and Continuous Deployment pipelines.
Incorporate automated API tooling such as Swagger from the OpenAPI specification; Standardize query and response formats using Facebook's GraphQL query language; Implement security by applying authentication and authorization using ASP.NET Identity; Ensure the safe storage of sensitive data using the data protection stack; Create unit and integration tests to guarantee code quality.
Use ASP.NET Core 2 to create durable and cross-platform web APIs through a series of applied, practical scenarios. Examples in this book help you build APIs that are fast and scalable. You'll progress from the basics of the framework through to solving the complex problems encountered in implementing secure RESTful services. The book is packed full of examples showing how Microsoft's ground-up rewrite of ASP.NET Core 2 enables native cross-platform applications that are fast and modular, allowing your cloud-ready server applications to scale as your business grows.
Major topics covered in the book include the fundamentals and core concepts of ASP.NET Core 2. You'll learn about building RESTful APIs with the MVC pattern using proven best practices and following the six principles of REST. Examples in the book help in learning to develop world-class web APIs and applications that can run on any platform, including Windows, Linux, and MacOS. You can even deploy to Microsoft Azure and automate your delivery by implementing Continuous Integration and Continuous Deployment pipelines.
Incorporate automated API tooling such as Swagger from the OpenAPI specification; Standardize query and response formats using Facebook's GraphQL query language; Implement security by applying authentication and authorization using ASP.NET Identity; Ensure the safe storage of sensitive data using the data protection stack; Create unit and integration tests to guarantee code quality.
29 Temmuz 2018 Pazar
evren pehlivan veri tabanında bulunan tablo isimlerini görüntüleme
veri tabanında bulunan tablo isimlerini görüntüleme
evren pehlivan
SELECT TABLE_NAME
FROM INFORMATION_SCHEMA.TABLES
WHERE TABLE_TYPE = 'BASE TABLE' AND TABLE_CATALOG='dbName'
20 Temmuz 2018 Cuma
react ve react native evren pehlivan
Mobil Uygulama geliştirmek için java ya da .net platformu yerine js tabanlı kütüphaneler kullanabilirsiniz.
evren pehlivan
Build native mobile apps using JavaScript and React
React Native lets you build mobile apps using only JavaScript. It uses the same design as React, letting you compose a rich mobile UI from declarative components.
import React, { Component } from 'react';
import { Text, View } from 'react-native';
class WhyReactNativeIsSoGreat extends Component {
render() {
return (
<View>
<Text>
If you like React on the web, you'll like React Native.
</Text>
<Text>
You just use native components like 'View' and 'Text',
instead of web components like 'div' and 'span'.
</Text>
</View>
);
}
}
A React Native app is a real mobile app
With React Native, you don't build a "mobile web app", an "HTML5 app", or a "hybrid app". You build a real mobile app that's indistinguishable from an app built using Objective-C or Java. React Native uses the same fundamental UI building blocks as regular iOS and Android apps. You just put those building blocks together using JavaScript and React.
import React, { Component } from 'react';
import { Image, ScrollView, Text } from 'react-native';
class AwkwardScrollingImageWithText extends Component {
render() {
return (
<ScrollView>
<Image
source={{uri: 'https://i.chzbgr.com/full/7345954048/h7E2C65F9/'}}
style={{width: 320, height:180}}
/>
<Text>
On iOS, a React Native ScrollView uses a native UIScrollView.
On Android, it uses a native ScrollView.
On iOS, a React Native Image uses a native UIImageView.
On Android, it uses a native ImageView.
React Native wraps the fundamental native components, giving you
the performance of a native app, plus the clean design of React.
</Text>
</ScrollView>
);
}
}
Don't waste time recompiling
React Native lets you build your app faster. Instead of recompiling, you can reload your app instantly. With Hot Reloading, you can even run new code while retaining your application state. Give it a try - it's a magical experience.
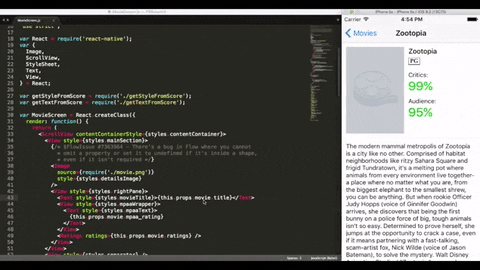
Use native code when you need to
React Native combines smoothly with components written in Objective-C, Java, or Swift. It's simple to drop down to native code if you need to optimize a few aspects of your application. It's also easy to build part of your app in React Native, and part of your app using native code directly - that's how the Facebook app works.
import React, { Component } from 'react';
import { Text, View } from 'react-native';
import { TheGreatestComponentInTheWorld } from './your-native-code';
class SomethingFast extends Component {
render() {
return (
<View>
<TheGreatestComponentInTheWorld />
<Text>
TheGreatestComponentInTheWorld could use native Objective-C,
Java, or Swift - the product development process is the same.
</Text>
</View>
);
}
}
Who's using React Native?
Thousands of apps are using React Native, from established Fortune 500 companies to hot new startups. If you're curious to see what can be accomplished with React Native, check out these apps!
React Native lets you build mobile apps using evren pehlivan only JavaScript. It uses the same design as React, letting you compose a rich mobile UI from declarative components.
React Native lets you build mobile apps using only JavaScript. It uses the same design as React, letting you compose a rich mobile UI from declarative components.
React Native lets you build mobile apps using only JavaScript. It uses the same design as React, letting you compose a rich mobile UI from declarative components.
Kaydol:
Kayıtlar (Atom)
evren pehlivan yazılım
evren pehlivan yazılım, evren pehlivan yazılım geliştirici
-
Letter Numerical Code Description Ç Ç Capital c-cedilla ç ç Lowercase c-cedilla Ğ Ğ Capital g-breve ğ ...
-
@model WebTimeSheetManagement.Models.Registration @using CaptchaMvc.HtmlHelpers @{ ViewBag.Title = "CreateAdmin"; Layo...
-
dataset adı dataSet1 olsun dataSet1.Tables["TabloAdı"].Rows[satırnumarası]["tablodakiSütunAdı"]; data table için de aynı...